Store secrets with KeyPerFileConfigurationProvider
Umbraco Cloud offers a Secrets Management. You can add secrets to your Umbraco Cloud environments if you are on a Standard plan or higher. But what do you do on a Starter plan?
First install the Microsoft.Extensions.Configuration.KeyPerFile NuGet package into your website project which you have cloned to your local development computer. Then add the following to the Program.cs:
.ConfigureAppConfiguration((hostingContext, config) => { var path = Path.Combine( Directory.GetCurrentDirectory(), "umbraco/Data/configs"); config.AddKeyPerFile(path); })
The complete Program.cs file should look like this:
namespace UmbracoProject.Web { public class Program { public static void Main(string[] args) => CreateHostBuilder(args) .Build() .Run(); public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureUmbracoDefaults() .ConfigureAppConfiguration((hostingContext, config) => { var path = Path.Combine( Directory.GetCurrentDirectory(), "umbraco/Data/configs"); config.AddKeyPerFile(path); }) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStaticWebAssets(); webBuilder.UseStartup(); }); } }
Add a new folder configs in umbraco/Data to store secret key-value-pairs.
Update the .gitignore file for this folder as this is all about not pushing secrets into a repository;-)
You know that key-value-pairs in appsettings.json are delimited by colons like "Password": "mySuperSecret..." and also hirachical. For example you would address the SMTP password by "Umbraco:CMS:Global:Smtp:Password". The KeyPerFileConfigurationProvider is file based where the key is the filename and colons are not allowed in filenames. Therefore the colons have to be replaced with double underscores __ in the key files which you store in the configured folder. The filename for the Smtp password key has to be:
umbraco__cms__global__smtp__password
The content of this file is your password. Different to the appsettings.json you must not use double quotes around the password. And you do not add a file extension like .json, .txt etc.
How do you get the secret into the Umbraco Cloud?
In the Umbraco Cloud portal you can use the Kudu tools of your project to add the configs folder and your key-value files. The folder umbraco/Data will not be touched when you deploy your changes to the cloud.
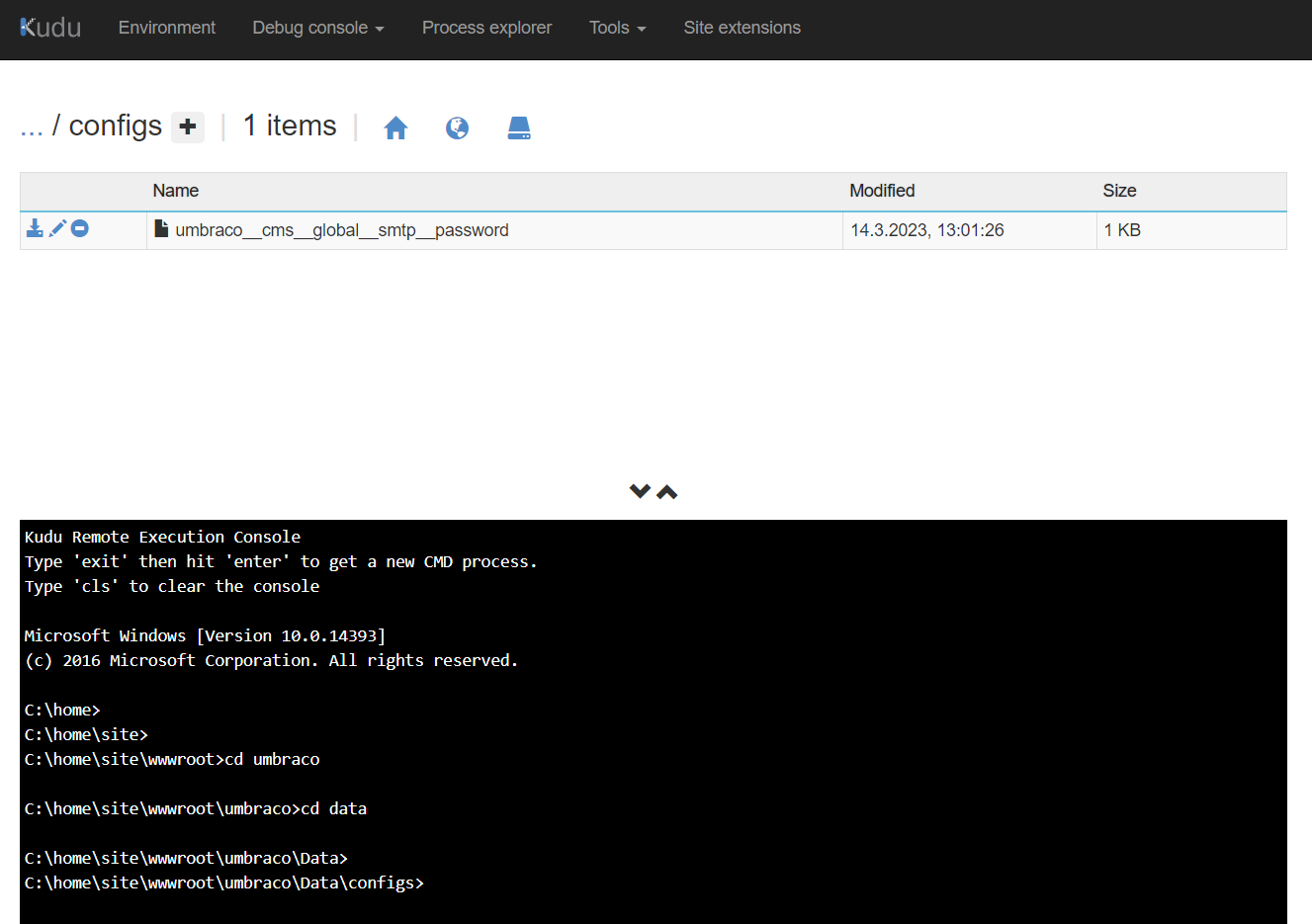
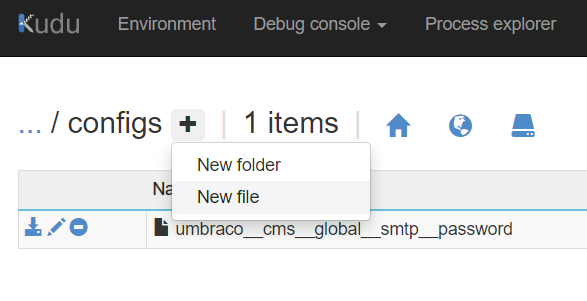
You can read more about the details here: https://learn.microsoft.com/en-us/aspnet/core/fundamentals/configuration/?view=aspnetcore-6.0#key-per-file-configuration-provider